API Reference / Examples Guidebook
RidgeRun Video Stabilization Library RidgeRun documentation is currently under development. |
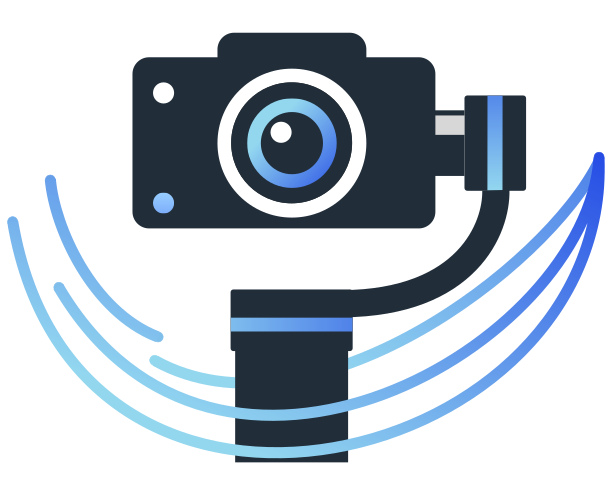
![]() |
![]() |
Introduction
This sections explains the details of how the code examples work.
Sensors
Bmi160 Example
To use the Bmi160 sensor the user must:
- Set the sensor settings and the sensor parameters.
- Create a Bmi160 instance and the Sensor Payload to save the results.
- Add the sensor usage logic.
Set the sensor settings and the sensor parameters
To set the sensor settings the user must establish its internal member: device_filename, which specifies the device bus where the sensor is mounted. While for the sensor parameters the user must establish the sensor's operating frequency (in Hz), the sample rate of the measurements (in Hz), and the sensor ID, which helps distinguish the connected sensors. Check the following pseudo-code as an example of it:
std::shared_ptr<rvs::SensorSettings> settings; std::shared_ptr<rvs::SensorParams> conf settings->device_filename = "/dev/i2c-7"; conf->frequency = 1; conf->sample_rate = 1; conf->sensor_id = 1;
Create a Bmi160 instance and the Sensor Payload
In order to use the sensor a Bmi160 Instance must be made. To do so, create a shared pointer of type ISensor and then use the Build method with kBmi160 and the settings as parameter. Also create a shared pointer of type SensorPayload to save later the results.
std::shared_ptr<rvs::ISensor> bmi160_sensor; std::shared_ptr<rvs::SensorPayload> payload; bmi160_sensor = rvs::ISensor::Build(rvs::Sensors::kBmi160, settings);
Sensor usage logic
Finally to use the sensor use the Start, Get and Stop method. Before getting data the sensor must be start, so start the sensor with the Start method by using the sensor parameters. After this, save the data on the payload variable defined on the last step with the Get method. When the sensor won't be needed anymore run the Stop method. Check the following code as example:
/* Starts the sensor */ bmi160_sensor->Start(conf); /* Starting usage logic */ /* Getting data */ bmi160_sensor->Get(payload); /* Ending usage logic */ /* Stops the sensor when it is not needed anymore */ bmi160_sensor->Stop();
Sample code
int main(int argc, char *argv[]) { std::shared_ptr<rvs::SensorSettings> settings; std::shared_ptr<rvs::SensorParams> conf std::shared_ptr<rvs::ISensor> bmi160_sensor; std::shared_ptr<rvs::SensorPayload> payload; settings->device_filename = "/dev/i2c-7"; conf->frequency = 1; conf->sample_rate = 1; conf->sensor_id = 1; bmi160_sensor = rvs::ISensor::Build(rvs::Sensors::kBmi160, settings); /* Starts the sensor */ bmi160_sensor->Start(conf); /* Starting usage logic */ /* Getting data */ bmi160_sensor->Get(payload); /* Use the results */ std::cout << payload->accel.x << std::endl; std::cout << payload->accel.y << std::endl; std::cout << payload->accel.z << std::endl; std::cout << payload->accel.timestamp << std::endl; std::cout << payload->gyro.x << std::endl; std::cout << payload->gyro.y << std::endl; std::cout << payload->gyro.z << std::endl; std::cout << payload->gyro.timestamp << std::endl; /* Ending usage logic */ /* Stops the sensor when it is not needed anymore */ bmi160_sensor->Stop(); return 0; }