RidgeRun Video Stabilization Library/API Reference/Adding New Sensors: Difference between revisions
(Created page with "<noinclude> {{RidgeRun Video Stabilization Library/Head|previous=API Reference/Library Architecture|next=API Reference/Adding Algorithm|metakeywords=imu,stabilizer,rb5,jetson,nvidia,xilinx,amd,qualcomm}} </noinclude> {{DISPLAYTITLE:API Reference / Adding New Sensors|noerror}} == Introduction == <noinclude> {{RidgeRun Video Stabilization Library/Foot|API Reference/Library Architecture|API Reference/Adding Algorithms}} </noinclude>") |
No edit summary |
||
Line 6: | Line 6: | ||
== Introduction == | == Introduction == | ||
The sensors are responsible for capturing the data needed to adjust the videos. Depending on the application, different types of IMU sensors may be required to measure the angular rate and orientation of objects. To add a new sensor, follow these steps: | |||
* Define the New Sensor class, inheriting from the ISensor class. | |||
* Define the Sensor Settings. | |||
* Define the Sensor Parameters. | |||
* Add the new sensor to Sensor Interface. | |||
== Define the New Sensor == | |||
The RidgeRun Sensor Interface, known as ISensor, is an extensible class designed to support various types of IMU sensors. To add a new sensor, the user must implement a new sensor class that derives from the ISensor class. This class must implement the following methods: | |||
* Start: Initializes and enables the sensor to begin data collection. | |||
* Stop: Stops the sensor. | |||
* Get: Retrieves data from the IMU sensor. | |||
=== Defining the Start method === | |||
This method receives a constant Sensor Parameter configuration. This configuration is conformed of the frequency that the sensor has to cover (in Hz), the sample rate of the measurements (in Hz) and the sensor id that helps to distinguish the number of the sensor connected. | |||
<syntaxhighlight lang=c++> | |||
RuntimeError NewSensorExample::Start( | |||
const std::shared_ptr<SensorParams> config) { | |||
RuntimeError ret{}; | |||
/* Sensor initialization logic */ | |||
return ret; | |||
} | |||
</syntaxhighlight> | |||
=== Defining the Stop method === | |||
This method ends disables the possibility to receive data from the sensor when it is called. It does not receive any parameter, so the logic to stop the sensor is managed internally. | |||
<syntaxhighlight lang=c++> | |||
RuntimeError NewSensorExample::Stop() { | |||
RuntimeError ret{}; | |||
/* Sensor stop logic */ | |||
return ret; | |||
} | |||
</syntaxhighlight> | |||
=== Defining the Get method === | |||
This method gets data from the IMU sensor. It receives a sensor payload parameter that is conformed of data from the accelerometer(m/s2), gyroscope (milligravitational) and magnetometer (if needed). The data of each of this is defined as: x-asis data, y-axis data and z-axis data, with its correspondent timestamp (in microseconds). The values received from the measurements must be saved on the payload to expose it to the user-space. | |||
<syntaxhighlight lang=c++> | |||
RuntimeError NewSensorExample::Get(std::shared_ptr<SensorPayload> payload) { | |||
RuntimeError ret{}; | |||
/* Get data logic */ | |||
/* Assign the accelerator data obtained */ | |||
payload->accel.x = accel_data_obtained.x; | |||
payload->accel.y = accel_data_obtained.y; | |||
payload->accel.z = accel_data_obtained.z; | |||
payload->accel.timestamp = accel_data_obtained.sensortime; | |||
/* Assign the gyroscope data obtained */ | |||
payload->gyro.x = gyro_data_obtained.x; | |||
payload->gyro.y = gyro_data_obtained.y; | |||
payload->gyro.z = gyro_data_obtained.z; | |||
payload->gyro.timestamp = gyro_data_obtained.sensortime; | |||
/* Assign the gyroscope data obtained (if measured) */ | |||
payload->mag.x = mag_data_obtained.x; | |||
payload->mag.y = mag_data_obtained.y; | |||
payload->mag.z = mag_data_obtained.z; | |||
payload->mag.timestamp = mag_data_obtained.sensortime; | |||
return ret; | |||
} | |||
</syntaxhighlight> | |||
<noinclude> | <noinclude> | ||
{{RidgeRun Video Stabilization Library/Foot|API Reference/Library Architecture|API Reference/Adding Algorithms}} | {{RidgeRun Video Stabilization Library/Foot|API Reference/Library Architecture|API Reference/Adding Algorithms}} | ||
</noinclude> | </noinclude> |
Revision as of 21:27, 28 June 2024
RidgeRun Video Stabilization Library RidgeRun documentation is currently under development. |
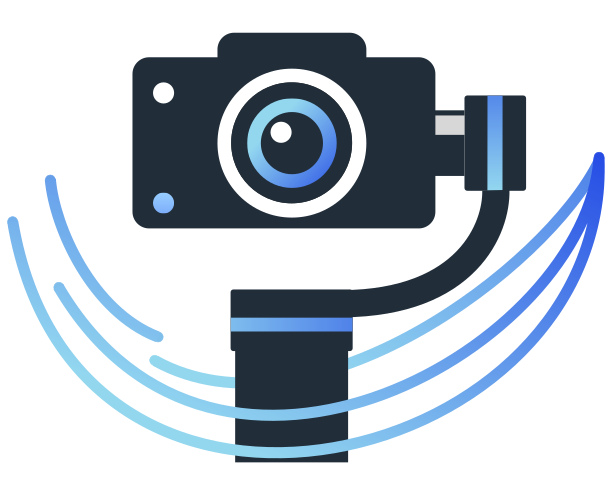
![]() |
![]() |
Introduction
The sensors are responsible for capturing the data needed to adjust the videos. Depending on the application, different types of IMU sensors may be required to measure the angular rate and orientation of objects. To add a new sensor, follow these steps:
- Define the New Sensor class, inheriting from the ISensor class.
- Define the Sensor Settings.
- Define the Sensor Parameters.
- Add the new sensor to Sensor Interface.
Define the New Sensor
The RidgeRun Sensor Interface, known as ISensor, is an extensible class designed to support various types of IMU sensors. To add a new sensor, the user must implement a new sensor class that derives from the ISensor class. This class must implement the following methods:
- Start: Initializes and enables the sensor to begin data collection.
- Stop: Stops the sensor.
- Get: Retrieves data from the IMU sensor.
Defining the Start method
This method receives a constant Sensor Parameter configuration. This configuration is conformed of the frequency that the sensor has to cover (in Hz), the sample rate of the measurements (in Hz) and the sensor id that helps to distinguish the number of the sensor connected.
RuntimeError NewSensorExample::Start( const std::shared_ptr<SensorParams> config) { RuntimeError ret{}; /* Sensor initialization logic */ return ret; }
Defining the Stop method
This method ends disables the possibility to receive data from the sensor when it is called. It does not receive any parameter, so the logic to stop the sensor is managed internally.
RuntimeError NewSensorExample::Stop() { RuntimeError ret{}; /* Sensor stop logic */ return ret; }
Defining the Get method
This method gets data from the IMU sensor. It receives a sensor payload parameter that is conformed of data from the accelerometer(m/s2), gyroscope (milligravitational) and magnetometer (if needed). The data of each of this is defined as: x-asis data, y-axis data and z-axis data, with its correspondent timestamp (in microseconds). The values received from the measurements must be saved on the payload to expose it to the user-space.
RuntimeError NewSensorExample::Get(std::shared_ptr<SensorPayload> payload) { RuntimeError ret{}; /* Get data logic */ /* Assign the accelerator data obtained */ payload->accel.x = accel_data_obtained.x; payload->accel.y = accel_data_obtained.y; payload->accel.z = accel_data_obtained.z; payload->accel.timestamp = accel_data_obtained.sensortime; /* Assign the gyroscope data obtained */ payload->gyro.x = gyro_data_obtained.x; payload->gyro.y = gyro_data_obtained.y; payload->gyro.z = gyro_data_obtained.z; payload->gyro.timestamp = gyro_data_obtained.sensortime; /* Assign the gyroscope data obtained (if measured) */ payload->mag.x = mag_data_obtained.x; payload->mag.y = mag_data_obtained.y; payload->mag.z = mag_data_obtained.z; payload->mag.timestamp = mag_data_obtained.sensortime; return ret; }