RidgeRun Video Stabilization Library/API Reference/Examples Guidebook: Difference between revisions
(Created page with "== Introduction == This sections explains the details of how the code examples work. == Sensors == === Bmi160 Example === To use the Bmi160 sensor the user must: * Set the sensor settings and the sensor parameters. * Create a Bmi160 instance and the Sensor Payload to save the results. * Add the sensor usage logic. ==== Set the sensor settings and the sensor parameters ==== To set the sensor settings the user must establish its internal member: device_filename, which...") |
No edit summary |
||
(5 intermediate revisions by 2 users not shown) | |||
Line 1: | Line 1: | ||
<noinclude> | |||
{{RidgeRun Video Stabilization Library/Head|previous=API Reference/Adding Backends|next=Library Integration for IMU|metakeywords=imu,stabilizer,rb5,jetson,nvidia,xilinx,amd,qualcomm}} | |||
</noinclude> | |||
{{DISPLAYTITLE:API Reference / Examples Guidebook|noerror}} | |||
== Introduction == | == Introduction == | ||
This | This section explains the details of how the code examples work. | ||
== Undistort == | |||
The undistort works as a test to verify the implementation of new accelerated undistort implementations. The code is available [http://ridgerun.pages.ridgerun.com/rnd/ridgerun-video-stabilizer/undistort_2undistort_8cpp-example.html here]. | |||
It requires OpenCV and OpenCL to work. The example performs a fixed transformation of a single image. You can test it by using the following command: | |||
<syntaxhighlight lang=bash> | |||
BUILD_DIR=./builddir | |||
IMAGE_PATH=$(pwd)/examples/undistort/4k_smpte.png | |||
${BUILDDIR}/examples/undistort/undistort ${IMAGE_PATH} | |||
</syntaxhighlight> | |||
It will save to images: | |||
* rvs_cv.png: results from the OpenCV backend | |||
* rvs_cl.png: results from the OpenCL backend | |||
The use of the undistort can be observed in [[RidgeRun_Video_Stabilization_Library/Library_Integration_for_IMU/Video_Undistortion|Video Undistortion]]. | |||
== Plotter == | |||
When analysing newer algorithms, it may be useful to create plots. You can find an example of the plotter in [http://ridgerun.pages.ridgerun.com/rnd/ridgerun-video-stabilizer/utils_2plotter-usage-example_8cpp-example.html this link]. | |||
The plotting support '''is not''' enabled by default. When compiling, please, make sure of installing the [[RidgeRun_Video_Stabilization_Library/Getting_Started/Building_the_Library#Optional|optional dependencies]] and compile with the meson option: <code>-Denable-plots=enabled</code>. | |||
After that, the example will be compiled. To run it, please: | |||
<syntaxhighlight lang=bash> | |||
BUILD_DIR=./builddir | |||
${BUILD_DIR}/examples/utils/plotter-usage-example | |||
</syntaxhighlight> | |||
The plotter API usage is the following: | |||
<syntaxhighlight lang=c++ line> | |||
rvs::Plotter<double> plotter{numpoints, {"series1", "series2"}}; | |||
std::unsorted_map<std::string, double> map; | |||
map["series1"] = 1.f; | |||
map["series2"] = 2.f; | |||
plotter.Plot(map); | |||
</syntaxhighlight> | |||
Line 1 creates a plotter instance compatible with double floating-point numbers. It will plot two series. | |||
Line 3 declares the map with the keys as the name of the series and the values, the values to plot. | |||
Line 5-6 assigns the values to the series. | |||
Line 8 plots a single point in the series. | |||
Important: the points are plotted as they are added by the <code>::Plot()</code> method. | |||
== Sensors == | == Sensors == | ||
Line 11: | Line 72: | ||
==== Set the sensor settings and the sensor parameters ==== | ==== Set the sensor settings and the sensor parameters ==== | ||
To set the sensor settings the user must establish its internal member | To set the sensor settings, the user must establish its internal member, device_filename, which specifies the device bus where the sensor is mounted. For the sensor parameters, the user must establish the sensor's operating frequency (in Hz), the sample rate of the measurements (in Hz), and the sensor ID, which helps distinguish the connected sensors. Check the following pseudo-code as an example of it: | ||
<syntaxhighlight lang=c++> | <syntaxhighlight lang=c++> | ||
Line 24: | Line 85: | ||
==== Create a Bmi160 instance and the Sensor Payload ==== | ==== Create a Bmi160 instance and the Sensor Payload ==== | ||
In order to use the sensor a Bmi160 Instance must be | In order to use the sensor, a Bmi160 Instance must be used. To do so, create a shared pointer of type ISensor and then use the Build method with kBmi160 and the settings as parameters. Also, create a shared pointer of type SensorPayload to save the results later. | ||
<syntaxhighlight lang=c++> | <syntaxhighlight lang=c++> | ||
Line 34: | Line 95: | ||
==== Sensor usage logic ==== | ==== Sensor usage logic ==== | ||
Finally to use the sensor use the Start, Get and Stop method. Before getting data the sensor must be | Finally, to use the sensor, use the Start, Get and Stop method. Before getting data, the sensor must be started, so start the sensor with the Start method by using the sensor parameters. After this, save the data on the payload variable defined in the last step with the Get method. When the sensor isn't needed anymore, run the Stop method. Check the following code as an example: | ||
<syntaxhighlight lang=c++> | <syntaxhighlight lang=c++> | ||
Line 92: | Line 153: | ||
</syntaxhighlight> | </syntaxhighlight> | ||
<noinclude> | |||
{{RidgeRun Video Stabilization Library/Foot|previous=API Reference/Adding Backends|next=Library Integration for IMU}} | |||
</noinclude> |
Latest revision as of 13:00, 19 August 2024
RidgeRun Video Stabilization Library RidgeRun documentation is currently under development. |
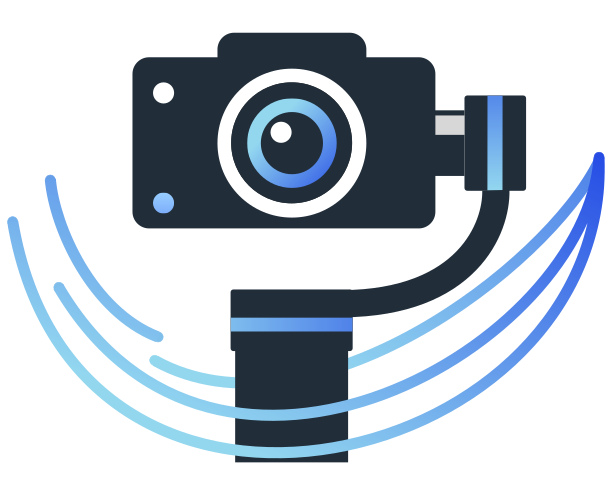
![]() |
![]() |
Introduction
This section explains the details of how the code examples work.
Undistort
The undistort works as a test to verify the implementation of new accelerated undistort implementations. The code is available here.
It requires OpenCV and OpenCL to work. The example performs a fixed transformation of a single image. You can test it by using the following command:
BUILD_DIR=./builddir IMAGE_PATH=$(pwd)/examples/undistort/4k_smpte.png ${BUILDDIR}/examples/undistort/undistort ${IMAGE_PATH}
It will save to images:
- rvs_cv.png: results from the OpenCV backend
- rvs_cl.png: results from the OpenCL backend
The use of the undistort can be observed in Video Undistortion.
Plotter
When analysing newer algorithms, it may be useful to create plots. You can find an example of the plotter in this link.
The plotting support is not enabled by default. When compiling, please, make sure of installing the optional dependencies and compile with the meson option: -Denable-plots=enabled
.
After that, the example will be compiled. To run it, please:
BUILD_DIR=./builddir ${BUILD_DIR}/examples/utils/plotter-usage-example
The plotter API usage is the following:
rvs::Plotter<double> plotter{numpoints, {"series1", "series2"}}; std::unsorted_map<std::string, double> map; map["series1"] = 1.f; map["series2"] = 2.f; plotter.Plot(map);
Line 1 creates a plotter instance compatible with double floating-point numbers. It will plot two series.
Line 3 declares the map with the keys as the name of the series and the values, the values to plot.
Line 5-6 assigns the values to the series.
Line 8 plots a single point in the series.
Important: the points are plotted as they are added by the ::Plot()
method.
Sensors
Bmi160 Example
To use the Bmi160 sensor the user must:
- Set the sensor settings and the sensor parameters.
- Create a Bmi160 instance and the Sensor Payload to save the results.
- Add the sensor usage logic.
Set the sensor settings and the sensor parameters
To set the sensor settings, the user must establish its internal member, device_filename, which specifies the device bus where the sensor is mounted. For the sensor parameters, the user must establish the sensor's operating frequency (in Hz), the sample rate of the measurements (in Hz), and the sensor ID, which helps distinguish the connected sensors. Check the following pseudo-code as an example of it:
std::shared_ptr<rvs::SensorSettings> settings; std::shared_ptr<rvs::SensorParams> conf settings->device_filename = "/dev/i2c-7"; conf->frequency = 1; conf->sample_rate = 1; conf->sensor_id = 1;
Create a Bmi160 instance and the Sensor Payload
In order to use the sensor, a Bmi160 Instance must be used. To do so, create a shared pointer of type ISensor and then use the Build method with kBmi160 and the settings as parameters. Also, create a shared pointer of type SensorPayload to save the results later.
std::shared_ptr<rvs::ISensor> bmi160_sensor; std::shared_ptr<rvs::SensorPayload> payload; bmi160_sensor = rvs::ISensor::Build(rvs::Sensors::kBmi160, settings);
Sensor usage logic
Finally, to use the sensor, use the Start, Get and Stop method. Before getting data, the sensor must be started, so start the sensor with the Start method by using the sensor parameters. After this, save the data on the payload variable defined in the last step with the Get method. When the sensor isn't needed anymore, run the Stop method. Check the following code as an example:
/* Starts the sensor */ bmi160_sensor->Start(conf); /* Starting usage logic */ /* Getting data */ bmi160_sensor->Get(payload); /* Ending usage logic */ /* Stops the sensor when it is not needed anymore */ bmi160_sensor->Stop();
Sample code
int main(int argc, char *argv[]) { std::shared_ptr<rvs::SensorSettings> settings; std::shared_ptr<rvs::SensorParams> conf std::shared_ptr<rvs::ISensor> bmi160_sensor; std::shared_ptr<rvs::SensorPayload> payload; settings->device_filename = "/dev/i2c-7"; conf->frequency = 1; conf->sample_rate = 1; conf->sensor_id = 1; bmi160_sensor = rvs::ISensor::Build(rvs::Sensors::kBmi160, settings); /* Starts the sensor */ bmi160_sensor->Start(conf); /* Starting usage logic */ /* Getting data */ bmi160_sensor->Get(payload); /* Use the results */ std::cout << payload->accel.x << std::endl; std::cout << payload->accel.y << std::endl; std::cout << payload->accel.z << std::endl; std::cout << payload->accel.timestamp << std::endl; std::cout << payload->gyro.x << std::endl; std::cout << payload->gyro.y << std::endl; std::cout << payload->gyro.z << std::endl; std::cout << payload->gyro.timestamp << std::endl; /* Ending usage logic */ /* Stops the sensor when it is not needed anymore */ bmi160_sensor->Stop(); return 0; }